Hey @CFL08 ,
I don’t have real data set at the moment but i created an example for you. Lets say you have flat surfaces at different depths.
import plotly.graph_objs as go
z1 = [
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4],
[4,4,4,4,4,4]
]
z2 = [[zij-1 for zij in zi] for zi in z1]
z3 = [[zij-2 for zij in zi] for zi in z1]
fig = go.Figure()
for step in range(3):
fig.add_trace(go.Surface(z=z1, visible=False, showscale=False)),
fig.add_trace(go.Surface(z=z2, visible=False, showscale=False)),
fig.add_trace(go.Surface(z=z3, visible=False, showscale=False)),
# Show First Layer
fig.data[0].visible = True
# Create slider
depth=[4,3,2]
steps = []
for i in range(3):
step = dict(method="update",
label = str(depth[i]),
args = [{"visible": [False] * len(fig.data)},
{"title": "Depth: " + str(depth[i])}
],
)
step["args"][0]["visible"][i] = True
steps.append(step)
sliders = [dict(active=0,
currentvalue={"prefix": "Depth: ", "suffix": " km"},
pad={"t": 50},
steps=steps)
]
#Button (Show All Traces)
fig.update_layout(updatemenus=[dict(type = "buttons", direction = "left",
buttons=list([dict(args=[{"visible":True}],
method="update",
label="Show All Traces"
)
]
),
pad={"r": 0, "t": 30},
showactive=True,
x=0.11,
xanchor="left",
y=1.1,
yanchor="top"
)
]
)
fig.update_layout(scene=dict(zaxis=dict(range=[1, 5],autorange=False)), sliders=sliders)
fig.show()
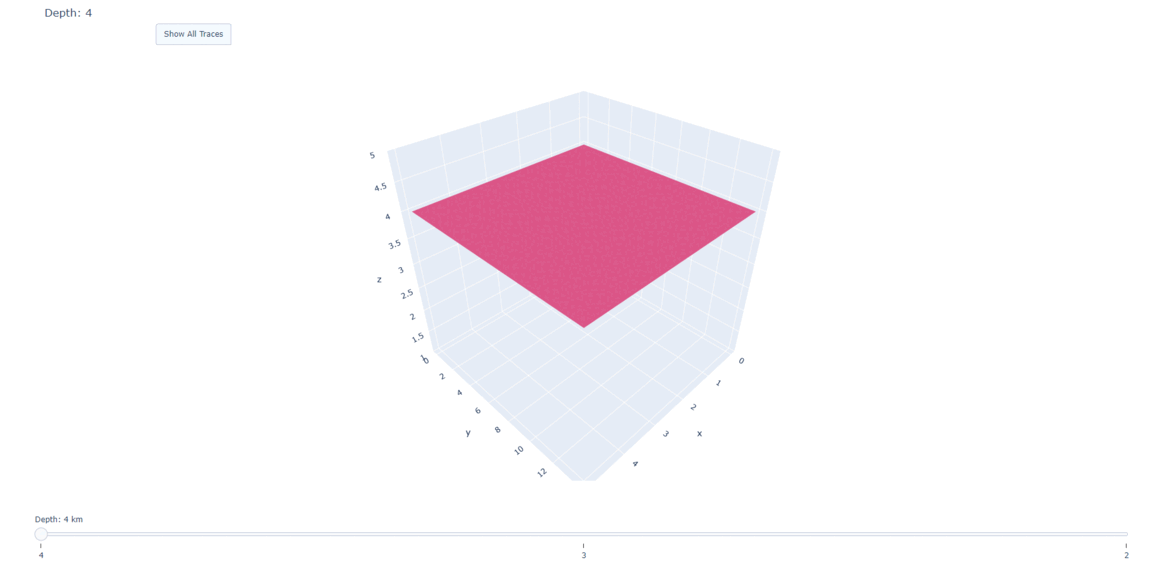
To create flat surface, lets say you have lon, lat, dat values.
fig = go.Figure()
fig.add_trace(go.Surface(x=lon, y=lat, z=np.ones(shape=lon.shape) * constant , # constant = your desired depth
surfacecolor = dat, # color corresponds to data
colorscale='viridis'
)
)
But I am not sure if we can create flattened 3D contours without filling.
If I discover something I’ll let you know, for dropdown menus and more functionality you can combine your plot with Dash.
Have a nice day.